Creation of entity type – Vertex AI Feature Store
Step 8: Creation of entity type
Entity type will be created under the newly created feature store using the create_entity_type method. Run the below-mentioned code in a new cell to create the entity type. The output of the last line in the code provides the path of the entity type created. Check the feature store landing page, the newly created feature store, and the entity type will be displayed:
entity_creation = client_admin.create_entity_type(
fs_s.CreateEntityTypeRequest(
parent=client_admin.featurestore_path(Project_id, location, featurestore_name),
entity_type_id=Entity_name,
entity_type=entity_type.EntityType(
description=”employee entity”,
),
)
)
print(entity_creation.result())
Step 9: Creation of feature
Once the feature store and entity type are created, the feature needs to be created before ingesting the feature values. For each of the features, information on feature ID, type, and description is provided. Run the following-mentioned code in a new cell to add features:
client_admin.batch_create_features(
parent=client_admin.entity_type_path(Project_id, location, featurestore_name, Entity_name),
requests=[
fs_s.CreateFeatureRequest(
feature=feature.Feature(
value_type=feature.Feature.ValueType.INT64,
description=”employee id”,
),
feature_id=”employee_id”,
),
fs_s.CreateFeatureRequest(
feature=feature.Feature(
value_type=feature.Feature.ValueType.STRING,
description=”education”,
),
feature_id=”education”,
),
fs_s.CreateFeatureRequest(
feature=feature.Feature(
value_type=feature.Feature.ValueType.STRING,
description=”gender”,
),
feature_id=”gender”,
),
fs_s.CreateFeatureRequest(
feature=feature.Feature(
value_type=feature.Feature.ValueType.INT64,
description=”no_of_trainings”,
),
feature_id=”no_of_trainings”,
),
fs_s.CreateFeatureRequest(
feature=feature.Feature(
value_type=feature.Feature.ValueType.INT64,
description=”age”,
),
feature_id=”age”,
),
],
).result()
Once the features are created, they are displayed in the output of the cell as shown in Figure 9.19:
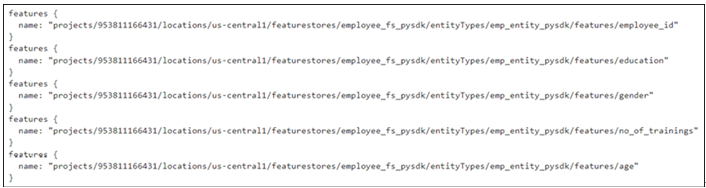
Figure 9.19: Addition of features to the feature store using Python
Step 10: Define the ingestion job
As seen in the web console, feature values can be ingested from cloud storage or BigQuery. We shall use the same CSV file which has been uploaded to the cloud storage. Importantly, we should also supply the timestamp information while ingesting the values. Timestamps can be provided in the code or there can be a separate column in the data that contains timestamp information. Timestamp information must be in google.protobuf.Timestamp format. Run the following code in a new cell to define the ingestion job:
seconds = int(datetime.datetime.now().timestamp())
timestamp_input = Timestamp(seconds=seconds)
ingest_data_csv = fs_s.ImportFeatureValuesRequest(
entity_type=client_admin.entity_type_path(
Project_id, location, featurestore_name, Entity_name
),
csv_source=io.CsvSource(
gcs_source=io.GcsSource(
uris=[
“gs://feature_store_input/employee_promotion_data_fs.csv”
]
)
),
entity_id_field=”employee_id”,
feature_specs=[
ImportFeatureValuesRequest.FeatureSpec(id=”employee_id”),
ImportFeatureValuesRequest.FeatureSpec(id=”education”),
ImportFeatureValuesRequest.FeatureSpec(id=”gender”),
ImportFeatureValuesRequest.FeatureSpec(id=”no_of_trainings”),
ImportFeatureValuesRequest.FeatureSpec(id=”age”),
],
feature_time=timestamp_input,
worker_count=1,
)
Note: If all feature values were generated at the same time, there is no need to have a timestamp column. Users can specify the timestamp as part of the ingestion request.
Step 11: Initiation of ingestion job
The ingestion job needs to be initiated after it is defined, run the following line of codes to begin the ingestion process:
ingest_data = client_admin.import_feature_values(ingest_data_csv)
ingest_data.result()
Once the ingestion is complete, it will provide information on the number of feature values ingested as shown in Figure 9.20:

Figure 9.20: Ingestion of feature values using Python